Authentication
To use the core APIs, access credentials to the platform are required. Credentials can be of two types: access tokens or a username/password pair. Additionally, the customer code (related to the environment), the environment code, and the refappId (reference app ID) must be provided, along with optionally a UUID and a user agent.
The authentication process generates a JWT token that has a duration of 15 minutes, plus an hour of inactivity during which the token is in a "RENEWABLE" state (it becomes active again upon first use).
Auth Flow
Below is the diagram representing the flow that returns a valid token to the client.
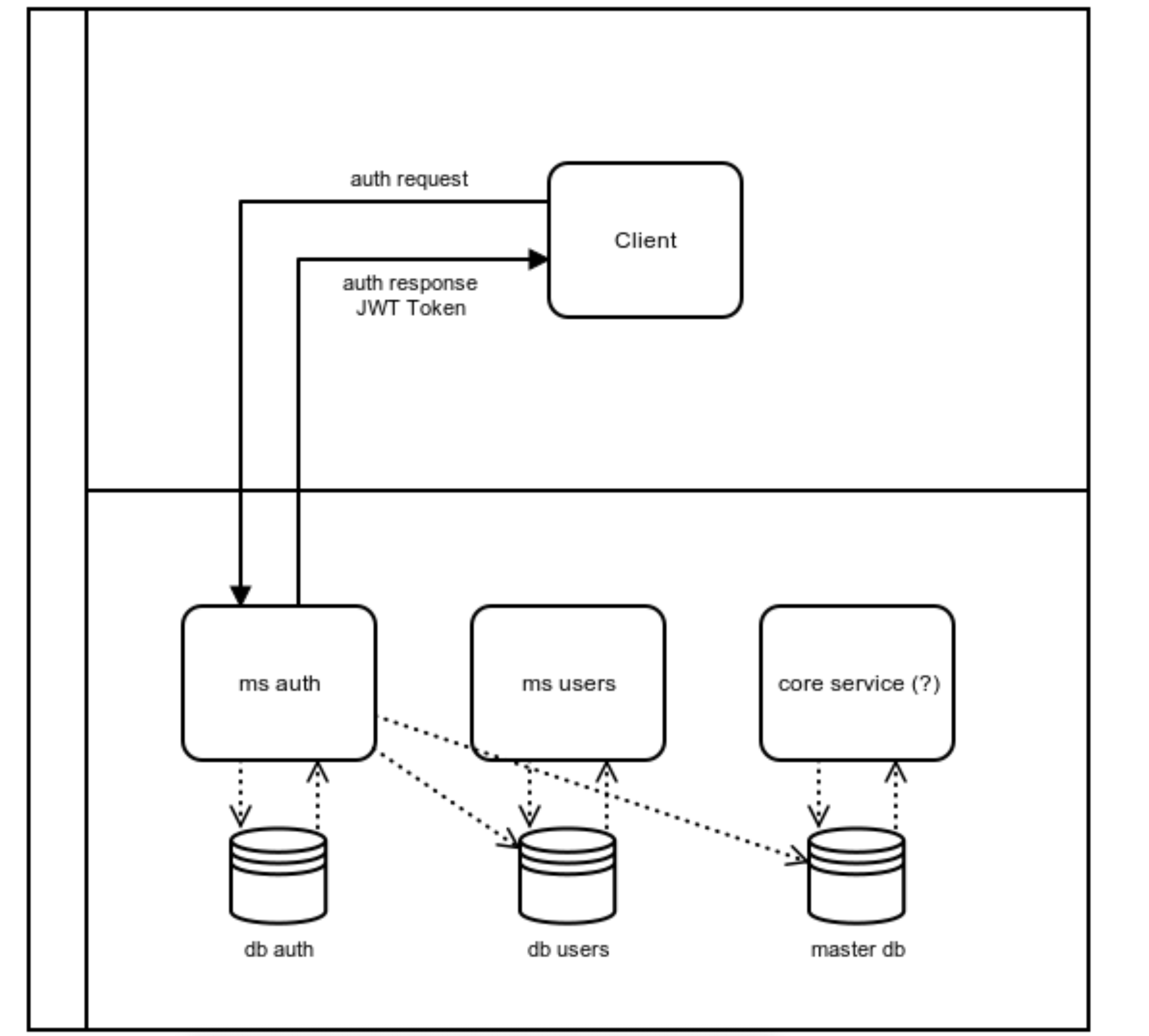
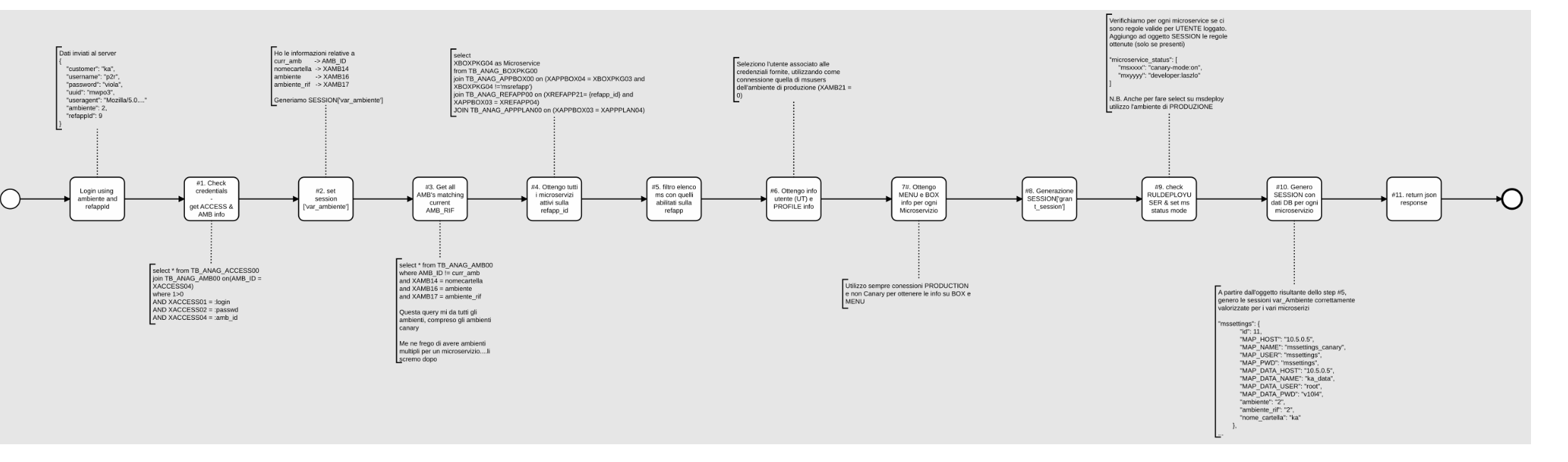
The authentication process consists of three phases:
-
Authentication: The provided credentials are verified in the microservice "msauth".
-
Profiling: User information linked to the provided credentials is retrieved, and the grants associated with the user are fetched.
-
Interface/Database Accessibility: Relevant information for generating interface menus (BOX and MENU) that the logged-in user can see is retrieved.
All retrieved data is saved in the session table, and the "sessionId"
is inserted into the generated JWT token.
JWT Token
An example of the obtained token is as follows
eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJpc3MiOiJwb3dlcjJyZXRhaWwuY29tIiwiYXVkIjoiaHR0cDpcL1wva2V0Y2hhcHAucG93ZXIycmV0YWlsLmNvbSIsImlhdCI6MTU4NTUwNzcyMCwibmJmIjoxNTg1NTA3NzIwLCJleHAiOjE1ODU1MDg2MjAsInNlc3Npb25JZCI6IjI2NiJ9PsXGIFRBCT7QpQycZQU6ZEm259xh8InPECTy-fIxkkmpR3f6Pz_zrLpYZAMwc4mjmWDeF-88Pb9XpLqLZdBxlo-wb0fDP7-LXNiT2cCeeDGkCPeBQaaLzOWSAdrVFdXvvcUYnZcTodPk0lFq2GINlCK-5D1QwOuvTs3nzpyLIYk
The clear content of the token is as follows
{
"iss": "q01.io",
"aud": "http://q01.io",
"iat": 1585507720,
"nbf": 1585507720,
"exp": 1585508620,
"sessionId": "266"
}
The "sessionId"
field, as described earlier, is the key to access the information (authorization and profiling) related to the owner of the token.
Login
The login endpoint is used to authenticate a user and obtain an authentication token.
Method
POST /api/v4/auth/login
Request Headers
key | value |
---|---|
content-type | application/json |
accept | application/json |
Request Body (raw json)
{
"username": "{{username}}",
"password": "{{password}}",
"resource": "{{resource}}",
"tenantId": "{{idTenant}}",
"uuid": "unique-device-id"
}
username
(string, required): The username of the user.password
(string, required): The password of the user.resource
(string, required): The resource being accessed.tenantId
(string, required): The ID of the tenant.uuid
(string, required): The UUID of the user.
it's possible to use key access_token
instead of username
and password
Response
Upon a successful authentication, the endpoint returns a JSON object with the following fields:
idToken
(string): The authentication token.issuedAt
(integer): The timestamp when the token was issued.durationTime
(string): The duration of the token validity.expiresIn
(integer): The expiration time of the token.
Here is an example of a Success response 200
{
"idToken": "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJpc3MiOiJxMDEuaW8iLCJhdWQiOiJxMDEuaW8iLCJpYXQiOjE3MTA1MTU5NTgsIm5iZiI6MTcxMDUxNTk1OCwiZXhwIjoxNzEwNTE2NTU4LCJzZXNzaW9uSWQiOjEyfQ.Zv2e62baUs9OrnrO7fPVPPAxpuRhpnD3jWiKYOCmouJgSfwcH-d5ooFgbDeEfXilI2KFkqzZA9iV-x8iXYr2_q_x2JZtVc76T07c1z33mL-VxezzZvCj1DxKmrWMDUDnp_kcUa7oCFOiERMp5k7d4ywWW1Puzg-fwubx6M3XJQE",
"issuedAt": 1710515958,
"durationTime": "600",
"expiresIn": 1710516558
}
and an Unauthorized response 401
{
"status": 0,
"message": "",
"data": [],
"errors": []
}
Logout
The logout endpoint is used to invalidate a session.
Method
GET /api/v4/auth/logout
Request Headers
key | value |
---|---|
content-type | application/json |
accept | application/json |
microservice | msauth |
authorization | bearer tokenId |
Response
status_code
(number): The status code of the response.message
(string, optional): A message related to the logout status.
An example of a Success response 200
{
"status_code": 200,
"message": ""
}
Token Validation
The token validation endpoint is used to validate an authentication token via an HTTP GET request
Method
GET /api/v4/auth/tokenvalidator
Request Headers
key | value |
---|---|
content-type | application/json |
accept | application/json |
microservice | msauth |
authorization | bearer tokenId |
Response
Upon a successful execution, the endpoint returns a JSON response with a status code of 200 and a content type of application/json. The response body includes the following key-value pairs:
code
: An integer representing the status code of the validation process.message
: A string providing additional information or error message related to the token validation.
Example of valid session
{
"code": 200,
"message": "Token is Valid"
}
Example of an invalid session
{
"code": 401,
"msg": "Invalid Auth Token provided. Can't access to the resource!",
"status": 401,
"message": "Invalid Auth Token provided. Can't access to the resource!"
}